- How To Make Simple Application Letter
- How To Make Simple App For Visual Studio Mac
- How To Make Simple Application For Visual Studio Mac Unity
This topic provides a step-by-step introduction to building, debugging, and publishing a simple .NET Core console application using C# in Visual Studio 2017. Visual Studio 2017 provides a full-featured development environment for building .NET Core applications. As long as the application doesn't have platform-specific dependencies, the application can run on any platform that .NET Core targets and on any system that has .NET Core installed.
Prerequisites
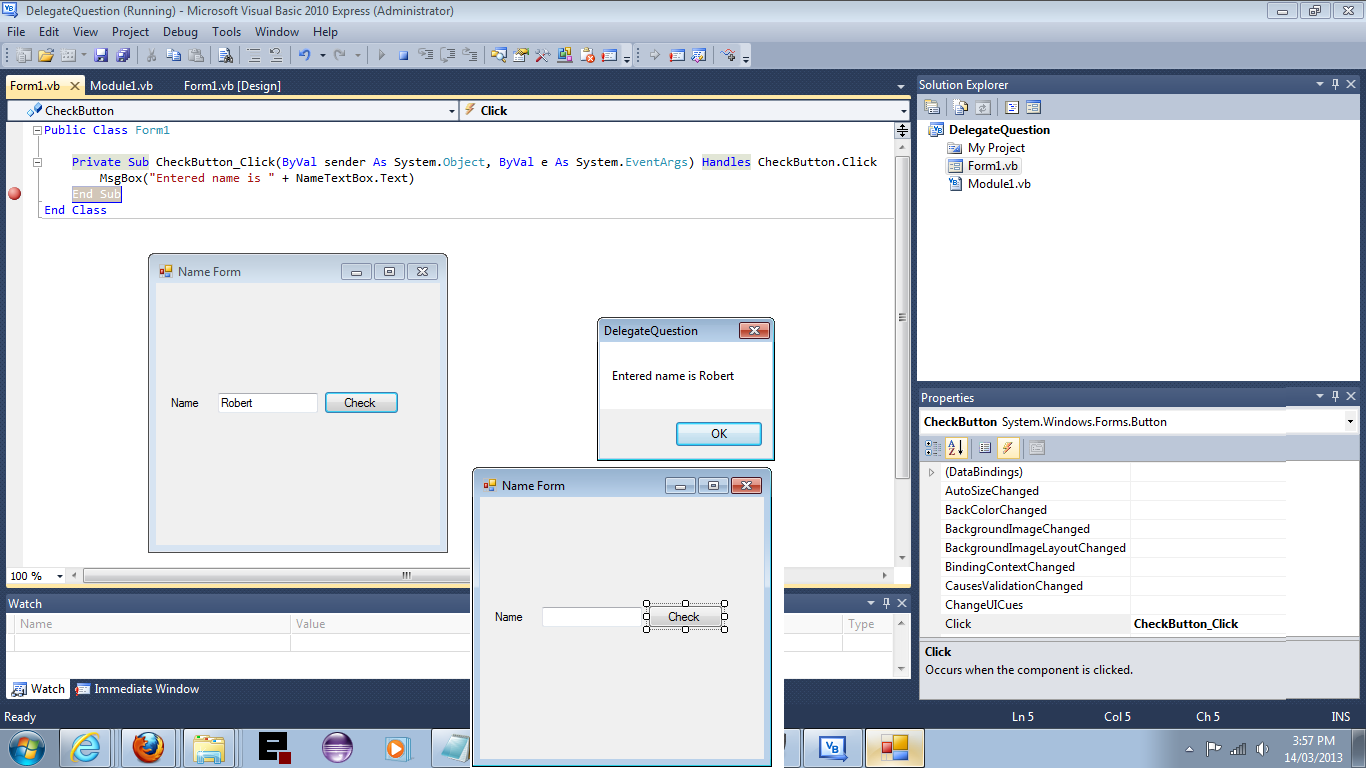
How To Make Simple Application Letter
The key here is that until you rewrite the application in a cross platform language, the application will have an odd look and feel in Mac OS X, but it will work (though it may require some tweaking). Testing your Xamarin app on iOS is a little trickier, because it requires a Mac to provide the emulator. If you have a Mac handy, follow the official instructions to set up the Mac agent and connect it to Visual Studio. My first thought would be to convert my application over to UWP, but I don't think there is an API to use this in Windows 8.x and 10. Alternatively I could use a borderless window that can open and close in a similar fashion to that menu, but it probably won't look as nice. Create an installer from Visual Studio The following article uses options that are available starting with the Freeware edition and project type. This tutorial will show you how to create installers for your Visual Studio solutions using Visual Studio 2017.
Visual Studio 2017 with the '.NET Core cross-platform development' workload installed. You can develop your app with either .NET Core 1.1 or .NET Core 2.0.
For more information, see the Prerequisites for .NET Core on Windows topic.
A simple Hello World application
Begin by creating a simple 'Hello World' console application. Follow these steps:
Launch Visual Studio 2017. Select File > New > Project from the menu bar. In the New Project* dialog, select the Visual C# node followed by the .NET Core node. Then select the Console App (.NET Core) project template. In the Name text box, type 'HelloWorld'. Select the OK button.
Visual Studio uses the template to create your project. The C# Console Application template for .NET Core automatically defines a class,
Program
, with a single method,Main
, that takes a String array as an argument.Main
is the application entry point, the method that's called automatically by the runtime when it launches the application. Any command-line arguments supplied when the application is launched are available in the args array.The template creates a simple 'Hello World' application. It calls the Console.WriteLine(String) method to display the literal string 'Hello World!' in the console window. By selecting the HelloWorld button with the green arrow on the toolbar, you can run the program in Debug mode. If you do, the console window is visible for only a brief time interval before it closes. This occurs because the
Main
method terminates and the application ends as soon as the single statement in theMain
method executes.To cause the application to pause before it closes the console window, add the following code immediately after the call to the Console.WriteLine(String) method:
This code prompts the user to press any key and then pauses the program until a key is pressed.
On the menu bar, select Build > Build Solution. This compiles your program into an intermediate language (IL) that's converted into binary code by a just-in-time (JIT) compiler.
Run the program by selecting the HelloWorld button with the green arrow on the toolbar.
Press any key to close the console window.
Enhancing the Hello World application
Enhance your application to prompt the user for their name and display it along with the date and time. To modify and test the program, do the following:
Enter the following C# code in the code window immediately after the opening bracket that follows the
static void Main(string[] args)
line and before the first closing bracket:This code replaces the contents of the
Main
method.This code displays 'What is your name?' in the console window and waits until the user enters a string followed by the Enter key. It stores this string into a variable named
name
. It also retrieves the value of the DateTime.Now property, which contains the current local time, and assigns it to a variable nameddate
. Finally, it uses an interpolated string to display these values in the console window.Compile the program by choosing Build > Build Solution.
Run the program in Debug mode in Visual Studio by selecting the green arrow on the toolbar, pressing F5, or choosing the Debug > Start Debugging menu item. Respond to the prompt by entering a name and pressing the Enter key.
Press any key to close the console window.
You've created and run your application. To develop a professional application, take some additional steps to make your application ready for release:
How To Make Simple App For Visual Studio Mac
For information on debugging your application, see Debug your .NET Core Hello World application using Visual Studio 2017.
For information on developing and publishing a distributable version of your application, see Publish your .NET Core Hello World application with Visual Studio 2017.
Related topics
How To Make Simple Application For Visual Studio Mac Unity

Instead of a console application, you can also build a class library with .NET Core and Visual Studio 2017. For a step-by-step introduction, see Building a class library with C# and .NET Core in Visual Studio 2017.
You can also develop a .NET Core console app on Mac, Linux, and Windows by using Visual Studio Code, a downloadable code editor. For a step-by-step tutorial, see Getting Started with Visual Studio Code.